The best way to
ship fastership fasterin TypeScript
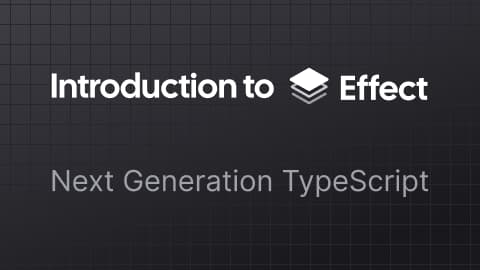
- Maximum Type-safety (incl. error handling)
- Makes your code more composable, reusable and testable
- Extensive library with a rich ecosystem of packages
- Clustering and Workflows (Alpha)
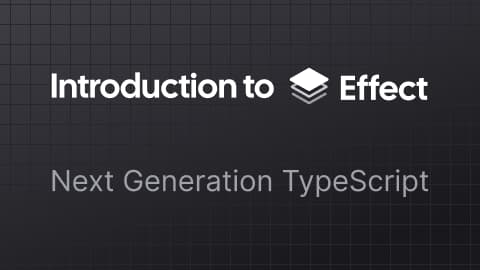
Effect works everywhere:
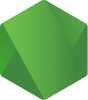
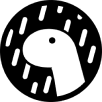
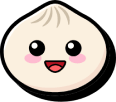
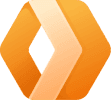
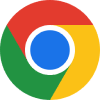
And with everything:
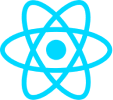
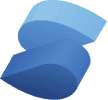
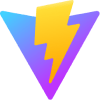
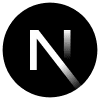
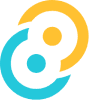
Production-grade TypeScript
As your application grows, Effect scales with it - keeping your code simple and maintainable.
Without Effect
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
async function getTodo(
id: number
): Promise<
| { ok: true; todo: any }
| { ok: false; error: "InvalidJson" | "RequestFailed" }
> {
try {
const response = await fetch(`/todos/${id}`)
if (!response.ok) throw new Error("Not OK!")
try {
const todo = await response.json()
return { ok: true, todo }
} catch (jsonError) {
return { ok: false, error: "InvalidJson" }
}
} catch (error) {
return { ok: false, error: "RequestFailed" }
}
}
With Effect
- 1
- 2
- 3
- 4
- 5
- 6
const getTodo = (
id: number
): Effect.Effect<unknown, HttpClientError> =>
httpClient.get(`/todos/${id}`).pipe(
Effect.andThen((response) => response.json)
)
The missing standard library for TypeScript
TypeScript/JavaScript, the most popular programming language, is still missing a standard library. Effect is filling this gap by providing a solid foundation of data structures, utilities, and abstractions to make building applications easier.
See 2022 State of JavaScript surveyPowerful building blocks
Every part of the Effect ecosystem is designed to be composable. The Effect primitives can be combined in many different ways to tackle the most complex problems.
- Immutable data structures
- Asynchronous queues & pub-sub
- Configuration & dependency management
No more one-off dependencies
With Effect the batteries are included. Regardless of the application, your package.json will have never been this small.
- Data validation & serialization
- Frameworks for CLI & HTTP applications
- Powerful abstractions for every platform
Never try & catch again
Effect doesn't shy away from errors — it embraces them as a fact of life. Successfully handle failure with the built-in error-handling primitives.
- Type-safe errors as values
- Powerful retry & recovery APIs
- Tools for logging & tracing
Let's see some example code
Doing the right thing in TypeScript is hard. Effect makes it easy!
We have collated some examples to show you how Effect can be utilized in your next project.
- Effect helps you with handling errors, async code, concurrency, streams and much more.
- Effect provides a unified replacement for many one-off dependencies.
- Effect integrates deeply with your current tech stack.
Effect helps you with handling errors, async code, concurrency, streams and much more.
Without Effect
- 1
- 2
- 3
- 4
- 5
const main = () => {
console.log('Hello, World!')
}
main()
Hello, World!
With Effect
- 1
- 2
- 3
- 4
- 5
import { Console, Effect } from 'effect'
const main = Console.log('Hello, World!')
Effect.runSync(main)
Hello, World!
Effect gives you new Superpowers
Composable & Reusable
Everything in the Effect ecosystem is designed to work together. Building applications with Effect feels like playing with Lego.
Maximum Type-Safety
Effect leverages the full power of TypeScript to give you confidence in your code. From errors to managing dependencies — if it compiles, it works.
Built-In Tracing
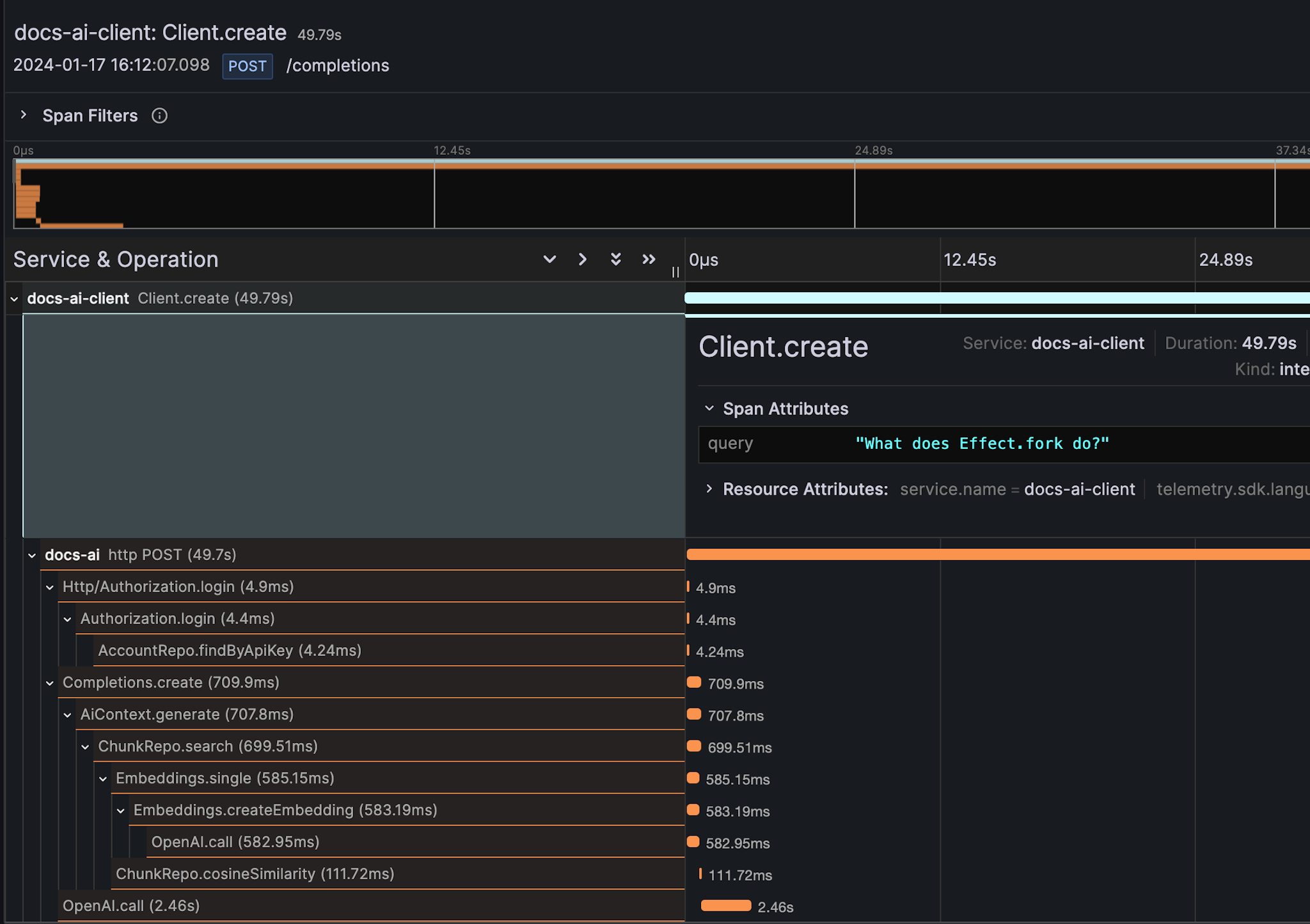
The built-in support for tracing is first-class. Effect integrates seamlessly with OpenTelemetry to give you full visibility over your application's performance.
Built-In Metrics
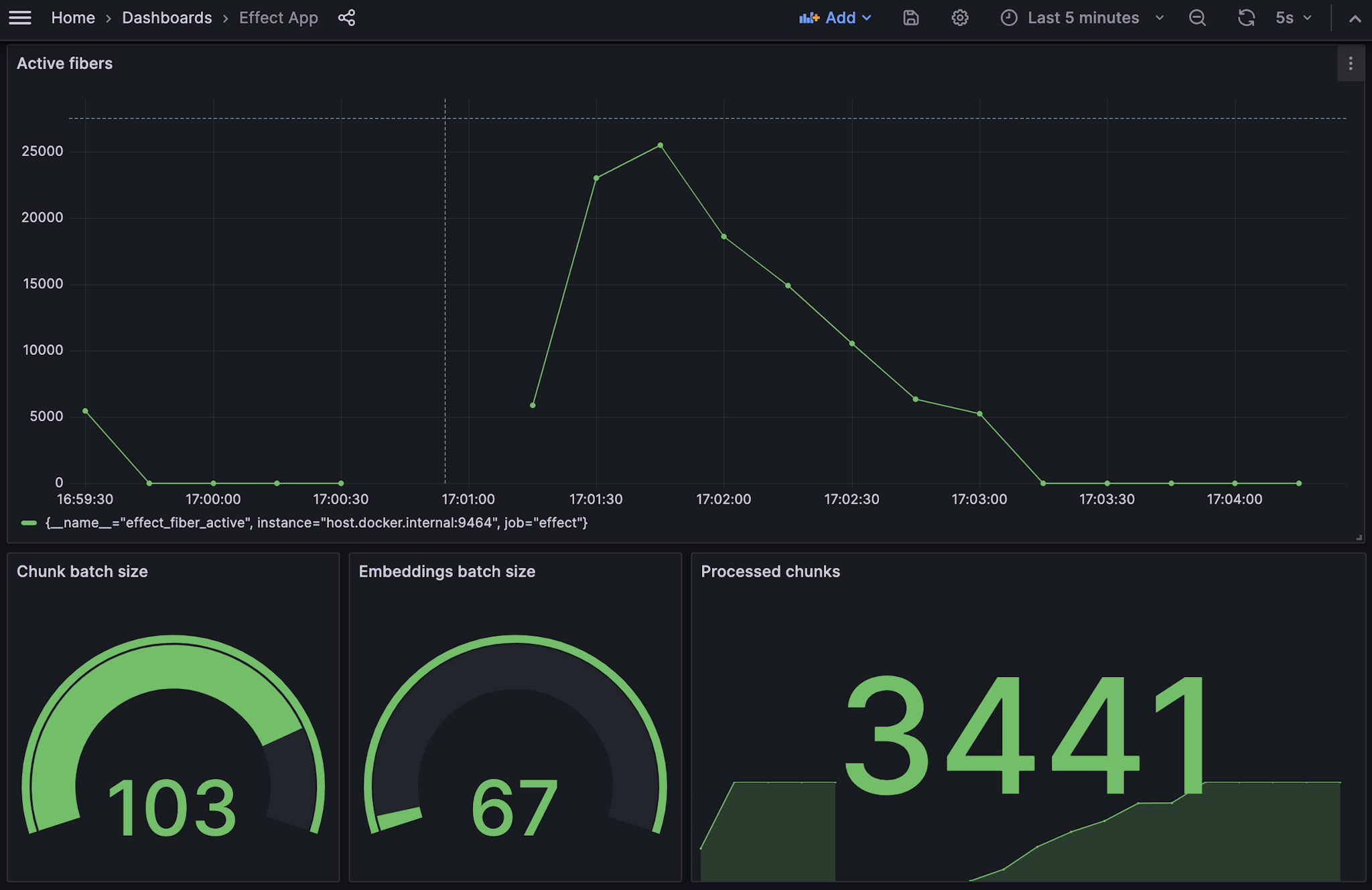
Effect has built-in support for metrics. You can use the provided OpenTelemetry exporter to integrate with a wide range of dashboards.
Easy to refactor
Make changes to your app with confidence. Effect's powerful abstractions make it easy to refactor your code without breaking anything.
What Effect users are saying
Effect is the 🐐 of TypeScript - Rust style error handling - Retries, Concurrency, Streams, … - Missing standard library
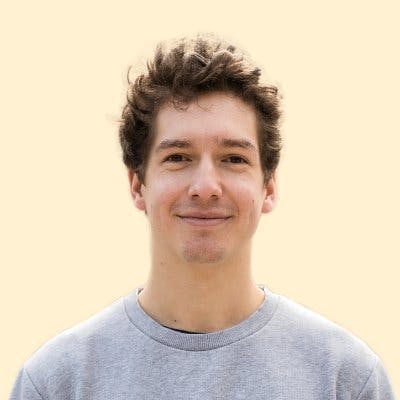
Effect is so, so good. Error handling in TypeScript has always felt so haphazard. Effect makes it feel effortless. And that’s only a tiny part of what makes Effect such a great set of libraries. Keep up the great work!
I LOVE Effect, been using it for a month now and it took a minute to figure out how to build composable services, but oh-my-god my code has never been this sexy.
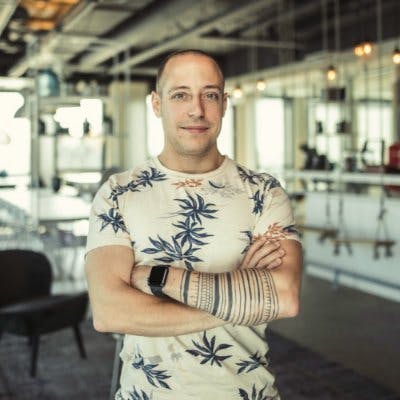
Delightfully, Effect is one of those rare tools that lift you up & educate you to become a better developer; Sustainably and well beyond the framework itself. And it does so both /effect/ively and *very* gently... Also, our community is *chefs kiss*
Okay, so what's the catch?
Learning curve
Learning Effect can be quite daunting but it doesn’t take long for you to be productive. It’s similar to learning TypeScript. It’s worth it.
Different programming style
To ensure a high level of type-safety, composabilty and tree-shakability, Effect's programming style might be different from what you're used to.
Extensive API surface
Effect has an API for every situation. While it may take some time to learn them all, a handful of the most common ones will get you a long way.
Frequently asked questions
Can I incrementally adopt Effect?
Yes! Adopting Effect you can start by refactoring small portions of your app, usually the ones with higher complexity, and keep going as you see fit
Does Effect scale?
Effect was built for production since the very beginning, we take good care of making everything as performant as possible, by providing you with better ways to deal with concurrency and great observability finding bottlenecks in your program becomes easy
Do I have to know functional programming?
No! While Effect makes usage of Functional Programming principles and patterns internally you can be proficient in Effect by simply using it as a smart Promise and forget that there is even a thing called Functional Programming
The library is huge, do I have to know it all?
No! Every module in Effect is made with a specific problem in mind that is deemed to be common enough but you don't need to know everything, in fact you can harness 80% of the productivity gain by just learning a few functions and 2-3 core modules.
What's the minimum bundle size?
The core of Effect is a runtime system that weights about 15k when compressed and tree-shaken, the rest scales with usage. If you end up using 100k of Effect code there is a good chance your app would have been 1Mb if not using Effect
Join our welcoming community
Start to
ship faster
in TypeScript
Effect makes it easy to build typed, robust & scalable applications. Check out our friendly documentation to get started.